/* Write a program that accepts two strings. * the program checks whether the second word is present anywhere in any word. * it the word is found then it prints the word from second string. * * An example could be: * Input : * Entr first string: this is a iss thiss. al a/ * Enter second string: is * output: this is iss thiss. */ /* * File: main.c * Author: umang * * Created on November 11, 2011, 3:08 PM */ #include <stdio.h> #include <stdlib.h> #include<string.h> #define true 0 #define false 1 //int main(int argc, char** argv) void main() { char arr[100] = {'\0'}; // initialize whole array char word[10] = {'\0'}; int i, j, temp; int b = false; printf("Enter string: "); gets(arr); printf("Enter word: "); gets(word); for (i = 0; arr[i] != '\0'; i++) { temp = i; b = false; for (j = 0; word[j] != NULL && arr[j + i] != NULL; j++) { if (word[j] != arr[i + j]) { b = true; } } if (b == false) { j--; if (arr[i + j] != ' ') { while (arr[i + j] != ' ' && arr[i + j] != NULL) { i++; } } while (arr[temp] != ' ' && temp >= 0) { temp--; if (temp < 0) { temp = 0; break; } } if (temp != 0) { temp++; } for (; arr[temp] != ' ' && arr[temp] != NULL; temp++) { printf("%c", arr[temp]); } printf(" "); i = temp; } } //return (EXIT_SUCCESS); } // input // this a te is thisi is. t // 4 12 18 22
Friday, November 11, 2011
string program in C
Saturday, October 1, 2011
no of coin problem
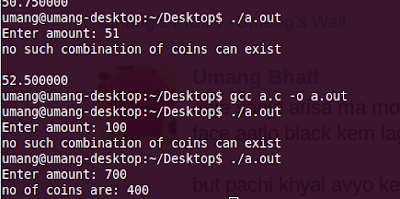
/*
rita has a money pouch containing Rsx (for example 700). There are equal number of 25 paise coins,
50 paise coins and one rupee coins. Write a C program to find out how many of each are there .
*/
/**************************
* author: umang bhatt *
* bhatt.umang7@gmail.com *
**************************/
/*
what i ubderstood:
0.25 x + 0.50 x + 1 x = amt
so dividing everything by 0.25 we get
x + 2x + 4x = amt * 4
therefore 7x = 4 amt
*/
#include<stdio.h>
void main()
{
int amt =700 ;
int i;
printf("Enter amount: " );
scanf("%d",&amt);
for( i = 1 ; i <= amt * 4 ; i++)
{
if( (7*i) == (4*amt) )
{
printf("no of coins are: %d\n",i );
break;
}
else if( (7*i) > (4*amt) )
{
printf("no such combination of coins can exist\n" );
break;
}
}
// printf("\n%f\n", ( (0.25*i) + (0.50 * i ) + ( i) ));
}
number operation
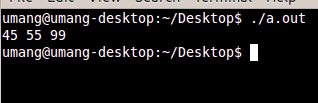
/************************** * author: umang bhatt * * bhatt.umang7@gmail.com * **************************/ /* consider following number 45 * 45 = 2025; 20+25 = 45. Write a program to generate number between 32 and 99 the satisfies the abovce property. */ #include<stdio.h> #define true 0 #define false 1 int foo(int n) { int i = n * n; int j ; int return_val; j = i % 100; i = i / 100; if((i+j)==n) { return_val = true; } else { return_val = false; } return return_val; } void main() { int i ; for( i = 32 ; i <= 99 ; i++) { if(foo(i) == true ) { printf("%d ",i ); } } printf("\n"); }
Labels:
20+25 = 45,
45 * 45 = 2025,
C program,
umang bhatt
Sunday, September 11, 2011
number program

/*
Definition from Aniruddha Guddi:
It can be seen that the number, 125874, and its double, 251748, contain exactly the same digits, but in a different order.
Find the smallest positive integer, x, such that 2x, 3x, 4x, 5x, and 6x, contain the same digits.
*/
/**************************
* author: umang bhatt *
* bhatt.umang7@gmail.com *
**************************/
#include<stdio.h>
#define TRUE 0
#define FALSE 1
int contains_same_digits(int no1, int no2)
{
int arr1[10] = {0};
int arr1_top = -1;
int arr2[10] = {0};
int arr2_top = -1;
int temp = no1;
int i, j, k;
int returnval;
int flag = FALSE;
//store numbers in array
while (temp > 0)
{
arr1[++arr1_top] = temp % 10;
temp = temp / 10;
}
temp = no2;
while (temp > 0)
{
arr2[++arr2_top] = temp % 10;
temp = temp / 10;
}
for (i = 0; i <= arr1_top; i++)
{
flag = FALSE;
for (j = 0; j <= arr2_top; j++)
{
if (arr1[i] == arr2[j])
{
flag = TRUE;
// remove that element from both arrays
for (k = i; k < arr1_top; k++)
{
arr1[k] = arr1[k + 1];
}
arr1_top--;
for (k = j; k < arr2_top; k++)
{
arr2[k] = arr2[k + 1];
}
arr2_top--;
if (j != 0)
j--;
}
}
if (flag == TRUE)
{
i = -1;
}
}
if (arr1_top == -1 && arr2_top == -1)
{
returnval = TRUE;
}
else
{
returnval = FALSE;
}
return returnval;
}
int main()
{
char c[5];
int i, j;
int flag;
int flag1;
for (j = 2; j <= 6; j++)
{
i = 1;
flag = FALSE;
while (flag == FALSE)
{
flag = contains_same_digits(i, (i * j));
if (flag == TRUE)
{
printf("\n %d * %d = %d where digits of %d and %d are same ", i, j, (i * j), i, (i * j));
flag = TRUE;
}
i++;
}
}
printf("\n");
return 0;
}
C program : largest palindrome made by multiplication of two 3 digit numbers
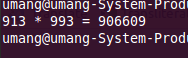
/*
Definition from Aniruddha Guddi:
A palindromic number reads the same both ways. The largest palindrome made from the product of two 2-digit numbers is 9009 = 91 × 99.
Find the largest palindrome made from the product of two 3-digit numbers.
*/
/**************************
* author: umang bhatt *
* bhatt.umang7@gmail.com *
**************************/
#include<stdio.h>
#define TRUE 0
#define FALSE 1
int is_palindrome(int no)
{
int temp = no;
int ans = 0;
int r;
while (temp > 0)
{
ans = ((ans * 10) + (temp % 10));
temp = temp / 10;
}
if (ans == no)
{
r = TRUE;
}
else
{
r = FALSE;
}
return r;
}
int main()
{
int f_no = -1;
int l_no = -1;
int last_palindrom = -1;
int flag = FALSE;
int i, j;
for (i = 100; i < 999; i++)
{
for (j = 100; j < 999; j++)
{
if (is_palindrome(i * j) == TRUE)
{
if ((i * j) > last_palindrom)
{
last_palindrom = i * j;
f_no = i;
l_no = j;
}
}
}
}
printf("%d * %d = %d \n", f_no, l_no, last_palindrom);
return 0;
}
Labels:
C,
C program,
is palindrome,
is_palindrome,
logic,
palindrome
Tuesday, May 3, 2011
factorial using stack
/****************************
* Umang B Bhatt *
* bhatt.umang7@gmail.com *
*****************************/
/**
* program for factorial using stack
*/
#include<stdio.h>
#include<string.h>
#define MAX 100
// push method
int push(int *s, int top, int ele)
{
int i;
if (top >= MAX)
{
printf("\nStack Overflow");
}
else
{
s[++top] = ele;
}
return top;
}
//pop method
int pop(int *a, int *top)
{
if ((*top) >= 0)
{
(*top) = (*top) - 1;
return a[(*top) + 1];
}
else
{
printf("Stack underflow\n");
return 0;
}
}
// personally, i believe that declaring TOP and S here is bad practice
void main()
{
int n;
int i; // loop variable
int ans = 1 ; // stroes the final answer
int TOP = -1; // stack variables that mainntain stack's top
int s[MAX]; // the stack
printf("\nEnter number: ");
scanf("%d",&n);
// here we can also make sure that the use is not entering a number
// that can not be accomodated in the stack
// if the user enters a number less than or equal to 0
// then we can not find factorial
if(n<=0)
{
printf("\nThe number can not be less than 0");
}
else
{
// push the numbers n,n-1 ....1 in the stack
// you can also go in reverse manner here. the result will be the same
// because in multiplication, order does not matter
for(i = n ; i >0 ; i--)
{
TOP = push(s,TOP, i);
}
// now pop all the elements one by one
// multiply them with the answer variable
while(TOP>=0)
{
ans = ans * pop(s,&TOP);
}
printf("\nFactorail is %d\n",ans);
}
// getch(); // will not work on linux
}
Labels:
C,
C program,
factorial,
factorial using stack
Sunday, April 17, 2011
program for odd even
/****************************
* Umang B Bhatt *
* bhatt.umang7@gmail.com *
*****************************/
/**
* program for odd even no / number
*/
#include<stdio.h>
int main(int argc, char *argv[])
{
int no;
printf("Enter a no to know whether a number is odd or even: ");
scanf("%d",&no);
if(no%2==0)
{
printf("the entered number %d is even\n",no);
}
else
{
printf("the entered number %d is odd\n",no);
}
//getch();
return 0;
}
Sunday, March 13, 2011
Pattern Program
/*
*
Enter an even number : 4
* * * *
* *
* *
* * * *
*/
/*
* No negative inputs please
*/
/**
* A quote: there are more than one ways to do it
*/
package javaapplication2;
import java.util.Scanner;
/**
*
* @author Umang
*/
public class Main
{
/**
* @param args the command line arguments
*/
public static void main(String[] args)
{
// loop variables
int j = 0, k = 0;
Scanner s = new Scanner(System.in);
System.out.print("Enter a number : ");
int n = s.nextInt();
System.out.print("\n");
for (j = 0; j < n; j++)
{
for (k = 0; k < n; k++)
{
if (k == 0 || k == n - 1 || j == 0 || j == n - 1)
{
System.out.print(" * ");
}
else
{
System.out.print(" ");
}
}
System.out.print("\n");
}
}
}
Pattern Program
/**
* user inputs a number greater than 2
*/
/**
run:
Enter an even number : 4
*
* *
* *
* *
* *
* *
*
*/
/*
* No negative inputs please
*/
/**
* A quote: there are more than one ways to do it
*/
package javaapplication2;
import java.util.Scanner;
/**
*
* @author Umang
*/
public class Main
{
/**
* @param args the command line arguments
*/
public static void main(String[] args)
{
boolean b = false;
// to print inner stars
int n1 = 0;
int n2 = 0;
// loop variables
int j = 0, k = 0;
Scanner s = new Scanner(System.in);
System.out.print("Enter an even number : ");
int n = s.nextInt();
n1 = n2 = n / 2 + 1;
System.out.print("\n");
for (j = 0; j <= n +2 ; j++)
{
for (k = 0; k < n * 2; k++)
{
if (k == n1 || k == n2)
{
System.out.print( " * " );
}
else
{
System.out.print(" ");
}
}
if(j-1==n/2)
{
b = true;
}
if (b == false)
{
n1--;
n2++;
}
else
{
n1++;
n2--;
}
System.out.print("\n");
}
}
}
Pattern Program
/********************************************************************
*i wrote this program when one great person was sitting with me :-)*
********************************************************************/
/*
Output:
Enter an Odd number : 5
* * * * *
* * *
* * * *
* * *
* * * * *
*/
// you can also do the same program using 3 loops
/*
* No negative inputs please
*/
/**
* A quote: there are more than one ways to do it
*/
package javaapplication2;
import java.util.Scanner;
/**
*
* @author Umang
*/
public class Main
{
/**
* @param args the command line arguments
*/
public static void main(String[] args)
{
boolean b = false;
// to print inner stars
int n1 = 0;
int n2 = 0;
// n1 and n2 indicate in which column the inner stars should be
// placed.
// in each iteration (of outer loop), the value of
// these two variables are changed
// loop variables
int j = 0, k = 0;
Scanner s = new Scanner(System.in);
System.out.print("Enter an Odd number : ");
int n = s.nextInt();
n1 = n2 = n / 2;
System.out.print("\n");
for (j = 0; j < n; j++)
{
for (k = 0; k < n; k++)
{
// if it is first or last line then print only *
// no spaces in first and last line
if (j == 0 || j == n - 1)
{
System.out.print(" * ");
}
else
{
// we come here if it is not first or last line
// n1 and n2 are the variables that hold informatin regarding where
// we want to print inner *s
// print * if it is the first or last column
// or the column value = n1 or n2
if (k == n1 || k == n2 || k == 0 || k == n - 1)
{
System.out.print(" * ");
}
else
{
// print blank space
System.out.print(" ");
}
}
}
// prnit new line
System.out.println();
// now change the place of inner *s
// this if changes the increment decrement pattern
if (j == (n / 2))
{
// if j == n / 2 then we need to change the pattern
// in which the numbers are incrementd
// b is a flag variable
b = true;
}
if (j != 0 && j != n - 1)
{
if (b == false)
{
// we come here if we are in first upper part of pattern
n1--;
n2++;
}
else
{
// we come here if we are in lower part of pattern
n1++;
n2--;
}
}
}
System.out.print("\n");
}
}
Tuesday, February 1, 2011
pattern

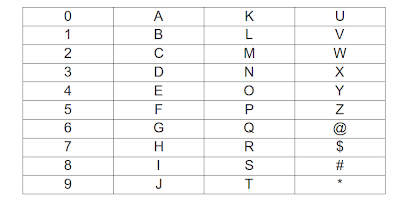
/**
* bhatt.umang7@gmail.com
* @author Umang B Bhatt
* if a number is repeated more than 3 times then exception should be thrown
*/
class MyException extends RuntimeException
{
public MyException(String message)
{
super(message);
}
}
public class test
{
public static void main(String args[])
{
if (args.length > 2)
{
// do something
System.out.print("Invalid number of arguments. More than one arguments were give.\nOnly onw was expected");
System.exit(0);
}
else if (args.length != 1)
{
System.out.print("You must pass some argument");
System.exit(0);
}
String st = args[0];
for (int i = 0; i < st.length(); i++)
{
if (Character.isDigit(st.charAt(i)) == false)
{
System.out.print("All the arguments needs to be numeric");
System.exit(0);
}
}
// now getting the array with values
char arr[][] = new char[10][3];
int val = 65;
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 10; j++)
{
arr[j][i] =(char) val;
val++;
}
}
arr[6][2] = '@' ;
arr[7][2] = '$' ;
arr[8][2] = '#' ;
arr[9][2] = '*' ;
for(int i = 0 ; i < st.length() ; i++)
{
char c = st.charAt(i);
int t = c -48;
if(arr[t][0]=='1')
{
throw new MyException("There were more than 3 accurance of same number");
}
else
{
System.out.print(arr[t][0]);
arr[t][0] = arr[t][1];
arr[t][1] = arr[t][2];
arr[t][2] = '1';
}
}
System.out.println();
}
}
Wednesday, January 19, 2011
pattern
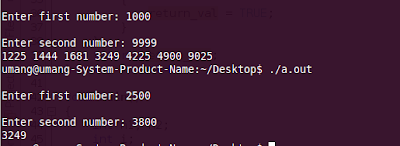
/****************************
* Umang B Bhatt *
* bhatt.umang7@gmail.com *
*****************************/
/*
* two numbers are entered. Both are of 4 digits.
* For each digit there can be three parts
* ( eg for 1234
* 1. 123 and 4
* 2. 12 and 34
* 3. 1 and 234)
*
* We need to find perfect suare number between first number and second number
* where both parts of any one part (of 3 parts) are also perfect square
*
*/
#include<stdio.h>
#define TRUE 1
#define FALSE 0
int perfectSquare(int n1)
{
long i;
int return_val = FALSE;
if (n1 == 1)
{
return TRUE;
}
for (i = 1; i < n1; i++)
{
if ((i * i) == n1)
{
return_val = TRUE;
}
}
return return_val;
}
void main()
{
int n1, n2;
int i;
int temp;
int div = 1000;
printf("\nEnter first number: ");
scanf("%d", &n1);
printf("\nEnter second number: ");
scanf("%d", &n2);
for (i = n1; i < n2; i++)
{
div = 1000;
while (div >= 10)
{
temp = i % div;
if (perfectSquare(temp) == TRUE)
{
temp = i / div;
if (perfectSquare(temp) == TRUE)
{
if (1 == perfectSquare(i))
{
printf("%d ", i);
}
}
}
div = div / 10;
}
}
printf("\n");
}
String pattern

/****************************
* Umang B Bhatt *
* bhatt.umang7@gmail.com *
*****************************/
/**
* program for string pattern
*/
/**
* Enter string: Please validate my Input
* Output: Jnpvt my vbljdbtf Plfbsf
*/
/**
* here all the words of a string are printed in reverse order (the last word comes first, second last wor comes second and so on)
* here the order of words in the word is not changed.
* whenever we encounter A, E , I , O or U replace it with the next character (eg A with B, E with F
* and so on)
* extra spaces in the program are also ignored
*/
#include<stdio.h>
#include<string.h>
void main()
{
char arr[100][100] = {0};
/* this trick also works with the structure
* we can initialize whole structure using this technique
*/
int x = 0, y = 0;
char str[100];
int i = 0;
printf("\nEnter string: ");
gets(str);
/*asusual warnings with gets*/
i = 0;
while (str[i] != '\0' && i < strlen(str))
{
if (str[i] == ' ' || str[i] == '\0')
{
while (str[i] == ' ' && str[i] != '\0')
{
i++;
}
}
else
{
if (str[i] == 'A' || str[i] == 'E' || str[i] == 'I' || str[i] == 'O' || str[i] == 'U' || str[i] == 'a' || str[i] == 'e' || str[i] == 'i' || str[i] == 'o' || str[i] == 'u')
{
arr[x][y] = str[i] +1 ;
y++;
i++;
}
else
{
arr[x][y] = str[i];
y++;
i++;
}
if (str[i] == ' ' || str[i] == '\0')
{
arr[x][y] = '\0';
x++;
y = 0;
}
}
}
printf("Output:");
for (i = x; i >= 0; i--)
{
printf(" %s", arr[i]);
}
printf("\n");
}
pattern
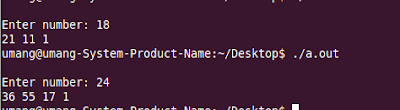
/****************************
* Umang B Bhatt *
* bhatt.umang7@gmail.com *
*****************************/
/**
* program for pattern
*/
/**
* input: 18
* output: 21 11 1
*
* 18 = 1 2 3 6 9
* now sum of 1 2 3 6 9 is 21
* 21 = 1 3 7
* now sum of 1 3 7 is 11
* 11 = 1
* // we stop at 1
*
*/
#include<stdio.h>
int fun(int n)
{
int sum = 0;
int i;
for (i = 1; i < n; i++)
{
if (n % i == 0)
{
sum = sum + i;
}
}
return sum;
}
void main()
{
int n;
int temp;
printf("\nEnter number: ");
scanf("%d", &n);
/* n is greater than 0*/
temp = n;
while (temp > 1)
{
temp = fun(temp);
printf("%d ", temp);
}
printf("\n");
}
pattern
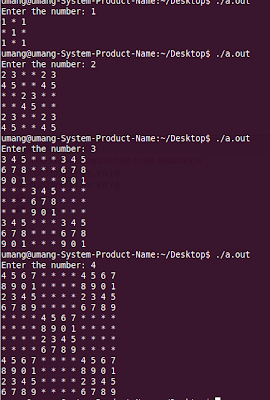
/****************************
* Umang B Bhatt *
* bhatt.umang7@gmail.com *
*****************************/
/**
* program for pattern
*/
#include<stdio.h>
void fun1(int arr[100][100], int n)
{
int i, j;
for (i = 0; i < n; i++)
{
for (j = 0; j < n; j++)
{
printf("%d ", arr[i][j]);
}
for (j = 0; j < n; j++)
{
printf("* ");
}
for (j = 0; j < n; j++)
{
printf("%d ", arr[i][j]);
}
printf("\n");
}
}
void fun2(int arr[100][100], int n)
{
int i, j;
for (i = 0; i < n; i++)
{
for (j = 0; j < n; j++)
{
printf("* ");
}
for (j = 0; j < n; j++)
{
printf("%d ", arr[i][j]);
}
for (j = 0; j < n; j++)
{
printf("* ");
}
printf("\n");
}
}
void main()
{
int arr[100][100] = {0};
/* this is a trick to assign all the values whether
they are float integer or char this will do all the appropriate assignments.
eg 0 to integer and long, 0.0f to float and null to a character
:refer iso standards
*/
int n;
int i, j;
int count;
printf("Enter the number: ");
/* the number should be between 1 to 9*/
scanf("%d", &n);
count = n;
for (i = 0; i < n; i++)
{
for (j = 0; j < n; j++)
{
arr[i][j] = count;
count++;
if (count > 9)
{
count = 0;
}
}
}
fun1(arr, n);
fun2(arr, n);
fun1(arr, n);
}
Subscribe to:
Posts (Atom)