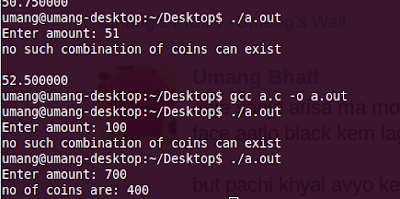
/*
rita has a money pouch containing Rsx (for example 700). There are equal number of 25 paise coins,
50 paise coins and one rupee coins. Write a C program to find out how many of each are there .
*/
/**************************
* author: umang bhatt *
* bhatt.umang7@gmail.com *
**************************/
/*
what i ubderstood:
0.25 x + 0.50 x + 1 x = amt
so dividing everything by 0.25 we get
x + 2x + 4x = amt * 4
therefore 7x = 4 amt
*/
#include<stdio.h>
void main()
{
int amt =700 ;
int i;
printf("Enter amount: " );
scanf("%d",&amt);
for( i = 1 ; i <= amt * 4 ; i++)
{
if( (7*i) == (4*amt) )
{
printf("no of coins are: %d\n",i );
break;
}
else if( (7*i) > (4*amt) )
{
printf("no such combination of coins can exist\n" );
break;
}
}
// printf("\n%f\n", ( (0.25*i) + (0.50 * i ) + ( i) ));
}