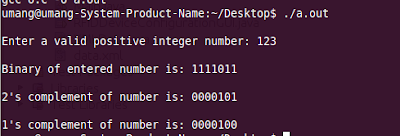
/*****************************
* Umang B Bhatt *
* bhatt.umang7@gmail.com *
*****************************/
/**
* program for decinal to binanry and 1's and 2's complement
*/
#include<stdio.h>
#include <string.h>
void main()
{
struct binary
{
int f : 2; // we use bit field here because it occupies less space
} b[100], c[100], d[100];
/**
* b[] will store binary, c[] will store 1's complement
* d[] will store 2's complement
*/
/* a will store decomal*/
long int a;
long int n, i, j; // n is the length of binary. i and j are for loops.
// now scanning pisitive number
do
{
printf("\nEnter a valid positive integer number: ");
scanf("%ld", &a);
}
while (a < 0);
for (i = 0; a != 0; i++)
{
b[i].f = a % 2;
a = a / 2;
}
n = i - 1;
printf("\nBinary of entered number is: ");
for (i = n; i >= 0; i--)
{
printf("%d", b[i].f);
}
/**
* 2's complement of binary nyumber
* binary : 1 0 1 0 1 1 0
* 2's complement: 0 1 0 1 0 1 0
* while going right to left put 0 as it is untill you get 1. put 1 as it is
* and then change 1 to 0 and 0 to 1.
*/
for (i = 0; i <= n; i++)
{
if (b[i].f == 1)
{
d[i].f = 1;
i++;
for (j = i; j <= n; j++)
{
if (b[j].f == 1)
{
d[j].f = 0;
}
else
{
d[j].f = 1;
}
}
break;
}
else
{
d[i].f = 0;
}
}
printf("\n\n2's complement of number is: ");
for (i = n; i >= 0; i--)
{
printf("%d", d[i].f);
}
/**
* getting and printing 1's complement
* binary : 1 0 1 0 1 1 0
* 1's complement : 0 1 0 1 0 0 1
* this one is having simple logic. change all 0 to 1 and all
* 1 to 0.
*/
printf("\n\n1's complement of number is: ");
for (i = n; i >= 0; i--)
{
if (b[i].f == 0)
{
c[i].f = 1;
// if it is 0 then change to 1
}
else
{
c[i].f = 0;
}
printf("%d", c[i].f);
}
printf("\n");
}
No comments:
Post a Comment